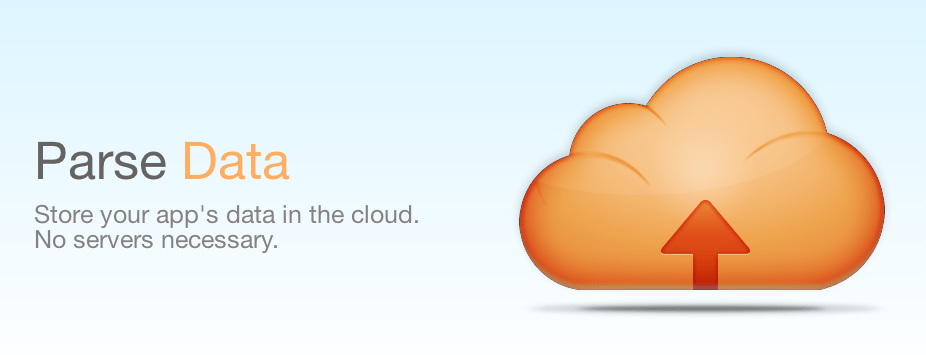
I’ve started using Parse.com API not more than a week ago, and I already fall in love with it. The first thing that hit me was “whoa, no extra work for storing objects in my database” and that’s really it. Saving data was never easier. Parse.com’s javascript API, the documentation is very good and it will help you to start your project in no time. Beside diving into the details, let me highlight another cool feature of Parse.com API. It’s the user management. Sign up someone, or let someone sign in, validate their email? Everything is there!
’nuff said, how to start?
Go to https://parse.com/apps, register a new application and copy the application key and javascript key.
Now open your html file, add the Parse.com’s js library
[sourcecode language=”html”]
<script type="text/javascript" src="http://www.parsecdn.com/js/parse-1.2.12.min.js"></script>
[/sourcecode]
and now initialize your Parse.com application
[sourcecode language=”javascript”]
Parse.initialize("Application ID", "Javascript Key");
[/sourcecode]
That’s it. Now you are ready to play with the rest of this article.
Sign me up, pronto!
One of the most tedious, boring work while developing an application is to deal with registration, email validation, ACL and taking care of the signing in process. With Parse.com API this is pretty straight forward. To sign up a new new user, all you gotta do is the following one!
[sourcecode language=”javascript”]
var user = new Parse.User();
user.set("username", "ElMacho");
user.set("password", "chicken");
user.set("email", "[email protected]");
user.signUp(null, {
success: function(user) {
// Everything’s done!
console.log(user.id);
},
error: function(user, error) {
alert("Error: " + error.code + " " + error.message);
}
});
[/sourcecode]
Your user is signed up! If you need to validate user’s email then log into your Parse.com dashboard and from your application’s settings section turn on the email validation. It’s simple.
Alright, let me in!
Alright, your users have signed up .Now let them get in. It’s simple to write a sign in script with Parse API
[sourcecode language=”javascript”]
Parse.User.logIn("ElMacho", "chicken", {
success: function(user) {
console.log(user.get("email"));
},
error: function(user, error) {
console.log("you shall not pass!");
}
});
[/sourcecode]
But hey, does an user have to login everytime? No, not at all. The logged in user’s state is saved in browser. So before attempting to sign in him/her again, you can check if he is already logged-in.
[sourcecode language=”javascript”]
var loggedInUser = Parse.User.current();
if(!loggedInUser){
//off you go, perform a log in
} else {
console.log(loggedInUser.get("email"));
}
[/sourcecode]
easy, eh?
Interesting, how can I deal with data?
Okay, enough with signing up and signing in. Lets save some data. Say, for example, we want to store user’s favorite books in Parse.com’s datastore. We will also retrieve the list later.
To save some data in Parse.com, we need to write code like this
[sourcecode language=”javascript”]
var user = Parse.User.current();
var Book = Parse.Object.extend("favoritebooks");
var b1 = new Book({"name":"King Solomon’s Mine","user":user});
b1.save();
var b2 = new Book({"name":"Return of She","user":user});
b2.save();
[/sourcecode]
While saving an object you can also take care of the error or success state.
[sourcecode language=”javascript”]
var b1 = new Book({"name":"King Solomon’s Mine","user":user});
b1.save(null, {
success:function(b){
console.log("successfully saved")
},
error:function(b,error){
console.log(error.message);
}
});
[/sourcecode]
You can save as many Books/data as you want, and link them up with the current user. Linking like this is useful for retrieving them in future. As we have a few saved records, lets pull them and see if they are really saved.
[sourcecode language=”javascript”]
var Book = Parse.Object.extend("favoritebooks");
var q = new Parse.Query(Book);
q.equalTo("user",user);
q.find({
success: function(results){
for(i in results){
var book = results[i];
console.log(book.get("name"));
}
}
});
[/sourcecode]
Once it runs, you can see that it outputs currently logged in user’s favorite books. Pretty neat, eh?
like equalTo, there are a dozen of useful constraints which you can use with your query. You can get the complete list at https://parse.com/docs/js_guide#queries-constraints . Parse.com’s documentation is pretty solid, and you will find almost everything over there.
It’s dinner time, log me out!
Simple, just call Parse.User.logOut() and you are done!
So is it just about user management and saving data?
No!, not at all!. But saving data and managing credentials + ACL are one of the coolest features that you can do with Parse.com API. Beside these object storage, you can host your application (ExpressJS based), you can run your code in the cloud (Background Job, Cloud Code), take care of the push notifications and even upload and store binary files.
In overall, Parse.com’s API is super cool. Give it a try today, you will love it for sure. And by the way, there is a super handy data browser in your application dashboard, use that!