if you are interested in developing twitter applications, you must have read about twitter API and it’s authentication protocol. your application can fetch user’s private data but it has to authenticate itself as the user for that. so there are two ways to do it
1. asking user to provide his twitter username and password to your application (well, i am not interested to give away my PASSWORD to anyone!!!)
2. let twitter handle the authentication on behalf of you and ask user to grant permission to your application (hmm!! interesting)
now you see that #2 is more safe for your user. and i think most security concerned users will choose this way. so your application have to initiate this type of authentication system using twittter’s supported authentication protocol oAuth (it’s a commonly used authentication protocol used among number of popular service providers like yahoo, google and others)
to implement oauth in php, the best way is to use an existing library. there are now numbers of libraries available for this purpose. following are some of them
1. oauth lib by andy smith
2. oauth library by marc worrell
3. oauth pecl extension by rasmus lerdorf and john jawed and felipe pena
now you see, pecl extensions are written in c and runs pretty faster. so i choose it without thinking much abt it. i have assumed that you know how to install a pecl extension in your php hosting and i am not going to blog detail about that right now. all that can help you right now is shell command “pecl install -f oauth” – you know, nothing talks better than command or code 🙂
after installing oauth extension in my hosting account, i start developing my twitter application. first i have to register my application with twitter. you can create your one by pointing your browser to http://twitter.com/oauth_clients/new. please remember that you have to provide a callback url which twitter use to redirect user of your application after a success/unsuccessful authentication. i will refer to that url as “callback_url” through out this blog post. my applications callback_url is “http://mydomain.tld/auth.php”
after you have done registering your application with twitter, it will give you the following important data.
1. consumer key
2. consumer secret
3. request token url
4. access token url
5. authorize url
you will be going to use all of these in your application. now lets see how oauth works in brief. it initiate the talk using your consumer key and secret key. and then it request the “request token” from the service provider. if u r successful, you have to forward user of your application to the “authorize url” with the “request token”. now the service provider will ask to grant permission to your application from the user. if user grants (or disagree) the permission, the service provider (here, twitter) will forward your user again to the “callback url” of your application with a “new token”. now with the help of this new token and the token grabbed from previous “request token” your application will ask for “access token”. once you have the access token, you can authorize you application as the user itself with same privilege.
lets see how to do it in php with the help of oauth pecl extension. here we are going to initiate the talk, get the token and forward user to the service provider’s authorizing url.
token.php
[sourcecode lang=”php”]
< ?php
//token.php
$oauth = new OAuth("consumer key","consumer secret",OAUTH_SIG_METHOD_HMACSHA1,OAUTH_AUTH_TYPE_URI); //initiate
$request_token_info = $oauth->getRequestToken(“http://twitter.com/oauth/request_token”); //get request token
file_put_contents(“token.txt”,$request_token_info[‘oauth_token_secret’]);//store the oauth token secret of request token
header(‘Location: http://twitter.com/oauth/authorize?oauth_token=’.$request_token_info[‘oauth_token’]);//forward user to authorize url
?>
[/sourcecode]
you see that we are storing the oauth_token_secret of the “request_token” because we need it in our next step to fetch access token. in the example above i am storing it in flat file, but you will have to store it in db/file with proper index to the userid so that you can retrieve it later in our next step.
if user visit this page, he will be redirected to twitter authorize url and that may look like the following one with different app name.
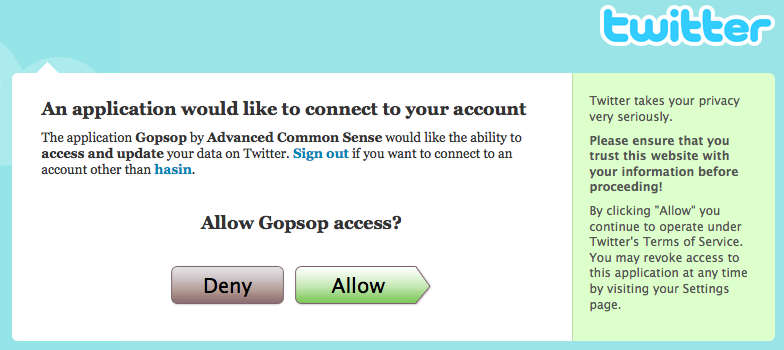
now lets see how we handle if the user click “allow” or “deny” in the above page.
this is the callback file you specified in settings of your app [auth.php]
[sourcecode lang=”php”]
< ?php
//auth.php
$oauth = new OAuth("consumer key","consumer secret",OAUTH_SIG_METHOD_HMACSHA1,OAUTH_AUTH_TYPE_URI); //initiate
$request_token_secret = file_get_contents("token.txt"); //get the oauth_token_secret of request token we stored previously
if(!empty($_GET['oauth_token'])){
$oauth->setToken($_GET[‘oauth_token’],$request_token_secret);//user allowed the app, so u
$access_token_info = $oauth->getAccessToken(‘http://twitter.com/oauth/access_token’);
}
?>
[/sourcecode]
access token is the most important token for your application. there are two object in this token – one is “oauth_token” and “oauth_token_secret”. if you print_r the access token it will look like the following one (actual value is not shown here)
Array (
[oauth_token] => abcdefg
[oauth_token_secret] => uvwxyz
)
you have to store this access token for authorizing later as this user (the user that was visiting). using this token you can anytime authorize yourself as that user and fetch user’s data from twitter. so lets see how we can fetch user’s profile data in rss (or json) format. the REST API url to fetch this data is “http://twitter.com/account/verify_credentials.json”. you can find other important REST urls to fetch user’s timeline, public timeline and friends timeline (also update status) in twitter’s documentation of it’s REST API
fetch user’s profile data
[sourcecode lang=”php”]
< ?php
//profile.php
$oauth = new OAuth("consumer key","consumer secret",OAUTH_SIG_METHOD_HMACSHA1,OAUTH_AUTH_TYPE_URI); //initiate
$oauth->setToken($accesstoken[‘oauth_token’],$accesstoken[‘oauth_token_secret’]);
$data = $oauth->fetch(‘http://twitter.com/account/verify_credentials.json’);
if($data){
$response_info = $oauth->getLastResponse();
echo “
";
print_r(json_decode($response_info));
echo "
“;
}
[/sourcecode]
the output of this code is the following one (my twitter username is hasin)
[sourcecode lang=”php”]
stdClass Object
(
[time_zone] => Dhaka
[friends_count] => 97
[profile_text_color] => 666666
[description] => Smoking too much PHP
[following] =>
[utc_offset] => 21600
[favourites_count] => 2
[profile_image_url] => http://s3.amazonaws.com/twitter_production/profile_images/84574185/afif_b_normal.jpg
[profile_background_image_url] => http://s3.amazonaws.com/twitter_production/profile_background_images/5565492/777481225666153.jpg
[profile_link_color] => 2FC2EF
[screen_name] => hasin
[profile_sidebar_fill_color] => 252429
[url] => http://hasin.wordpress.com
[name] => hasin
[protected] =>
[status] => stdClass Object
(
[text] => ok, understood how twitter auth works via oauth pecl ext. of #php. thanks to @rasmus for his excellent example
[in_reply_to_user_id] =>
[favorited] =>
[in_reply_to_screen_name] =>
[truncated] =>
[created_at] => Sat May 02 16:08:28 +0000 2009
[id] => 1679349376
[in_reply_to_status_id] =>
[source] => web
)
[profile_sidebar_border_color] => 181A1E
[profile_background_tile] => 1
[notifications] =>
[statuses_count] => 1147
[created_at] => Fri Nov 09 10:40:14 +0000 2007
[profile_background_color] => 1A1B1F
[followers_count] => 265
[location] => Dhaka, Bangladesh
[id] => 10094392
)
[/sourcecode]