Graph API from Facebook is a very interesting project based on open graph protocol. Facebook is gradually setting Graph API as the standard and deprecating the use of their old REST based APIs. To start working with Graph API doesnt need an extensive knowledge on how Facebook API works but its always good to have a sound knowledge on that. Graph API uses oAuth based authentication mechanism and using the auth token, they can identify who is making the API calls. I have been working on Facebook events using Graph API for last couple of days and I will be sharing my experience in this series of two or three posts where I am going to cover the following topics
1. Authenticating a user
2. How to create a Facebook Event with picture
3. How to post on Events Wall
4. How to manage users
5. How PHP SDK can be a life saver in some cases 🙂
Authenticating a user
Its very important that you know how to authenticate an user against Facebook and ask for appropriate permissions to be granted. To work with public events in Facebook, you need to ask for “create_event” permission from your visitors. Otherwise, in case of private events, you need to be granted permission for “user_events” and “friend_events”. For now, lets consider that we will be creating public events and we will ask for only “create_event” permission.
Lets create a new application in Facebook which will point to our working url. The following screenshot will show you which informations are necessary to create such an app. Once you are done, save the API_KEY/APP_ID and SECRET_KEY safe in somewhere for later use.
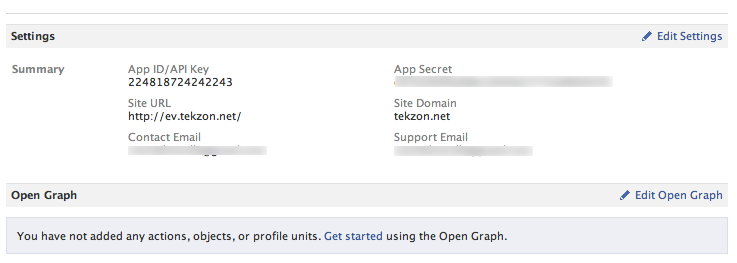
Now you are done with creating a Facebook app, its time to code 🙂 The following codeblock will prompt any visitor to log in with Facebook and grant the application some permissions. We are using our JS-SDK. We are also saving the auth token. You will soon find out why that would be necessary.
[sourcecode language=”javascript”]
<!html>
<head>
<title>My Facebook Event App</title>
<script src=’http://ajax.googleapis.com/ajax/libs/jquery/1.6.4/jquery.min.js’></script>
</head>
<body>
Welcome to our New Facebook App <span id=’fbinfo’><fb:name uid=’loggedinuser’ useyou=’false’></fb:name></span>
<div id=’fb-root’></div>
<script type="text/javascript" src="http://connect.facebook.net/en_US/all.js"></script>
<script type=’text/javascript’>
var fbuserid, fbtoken;
var appid = "224818724242243";
var loggedin = false;
$(document).ready(function() {
//loginFB();
});
FB.init({appId: appid, status: true, cookie: true, xfbml: true});
FB.Event.subscribe(‘auth.sessionChange’, function(response) {
if (response.session) {
var session = FB.getSession();
fbtoken = session.access_token;
fbuserid = session.uid;
}
});
FB.getLoginStatus(function(response) {
if (response.session) {
var session = FB.getSession();
fbtoken = session.access_token;
fbuserid = session.uid;
}
else{
loginFB();
}
});
function loginFB() {
FB.login(function(response) {
if (response.session) {
var session = FB.getSession();
fbtoken = session.access_token;
fbuserid = session.uid;
}
}, {perms:’create_event’});
}
function logoutFB() {
FB.logout(function(response) {
// user is now logged out
});
}
</script>
</body>
</html>
[/sourcecode]
Now when you visit your application url, for example http://ev.tekzon.net in our case, you will be prompted an authentication windows like this
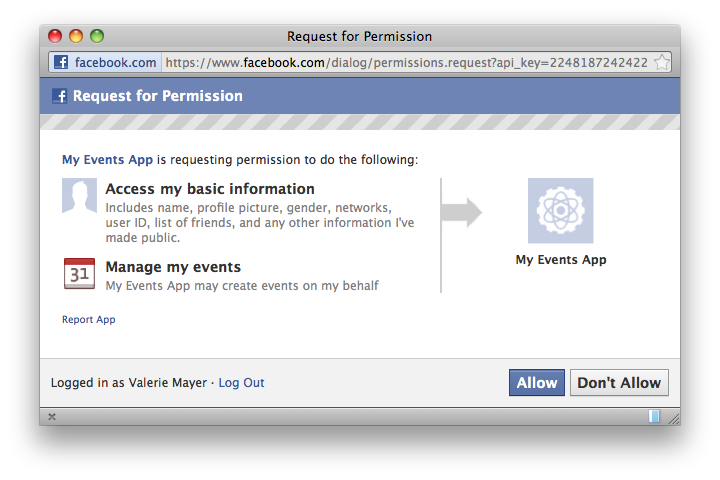
So now users are successfully authenticated to our new Facebook application, lets move to the next part
Creating a Facebook event using JS-SDK
To create a Facebook event, we need to pass the following parameters to “/me/events” graph url.
- name: Name of the event
- start_time: Start time of the event in UNIX timestamp format, or in “mm/dd/yyyy H:i” format 🙂
- end_time: End time of the event in UNIX timestamp format, or in “mm/dd/yyyy H:i” format 🙂
- location: Location of the event, as a string
- description: Description of the event
- picture: Profile picture for the event, we can not use that with JS SDK.
- privacy: either “OPEN” or “PRIVATE”, we will use “OPEN” in this example
So, here is a Javascript function which can create this event. In the following codeblock you will find a wrapper javasript function “createEvent()” to create this event and an example function to use that createEvent() function
[sourcecode language=”javascript”]
function createEvent(name, startTime, endTime, location, description) {
var eventData = {
"access_token": fbtoken,
"start_time" : startTime,
"end_time":endTime,
"location" : location,
"name" : name,
"description":description,
"privacy":"OPEN"
}
FB.api("/me/events",’post’,eventData,function(response){
if(response.id){
alert("We have successfully created a Facebook event with ID: "+response.id);
}
})
}
function createMyEvent(){
var name = "My Amazing Event";
var startTime = "10/29/2011 12:00 PM";
var endTime = "10/29/2011 06:00 PM";
var location = "Dhaka";
var description = "It will be freaking awesome";
createEvent(name, startTime,endTime, location, description);
}
[/sourcecode]
Now once you invoke this “createMyEvent()” function, it will show you the event id of your newly created event, if successful. Lets see how that would look like.
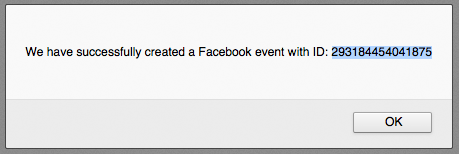
Ok, we can see that the event id of our newly created event is “253320941378247” – we can check it out in Facebook by going to http://facebook.com/events/253320941378247 – Meanwhile we can also check this same event using open graph protocol by going to http://graph.facebook.com/253320941378247
Ok, so now we know how to create a Facebook Event using JS-SDK and Graph API. But there is a major problem in creating a Faceboko event using JS-SDK and that is, you cannot attach any Profile Picture with this event. And you have to use a server side SDK (we will be using PHP-SDK in the following example) to do that. Lets see how can we create a Facebook event with a profile picture.
Creating a Facebook event with Profile Picture
We need to post the event parameters (use Ajax) to our server side script. Lets assume that the image file we are planning to use as the Profile Picture for our Facebook event resides in “/path/to/my/image.jpg”.
Here is a very important information for you and that is such an image’s minimum width MUST BE 100px – There is no documentation on that, and apparently I had to waste almost 4 hours to find what is the problem with my code. I just wish Facebook API documentation were better mentioning such things which may lead developers to waste huge time.
Ok, in the following codeblock, we will be using a new javascript function “createMyEventWithPHP()” which will post the event data to our server side php script event.php – Before that, download the PHP-SDK of Facebook from http://github.com/facebook/php-sdk, unzip and place in the same path where you are keeping this “events.php”
[sourcecode language=”javascript”]
function createEventWithPHP(name, startTime, endTime, location, description) {
var eventData = {
"access_token": fbtoken,
"start_time" : startTime,
"end_time":endTime,
"location" : location,
"name" : name,
"description":description,
"privacy":"OPEN"
}
$.post("/events.php",eventData,function(response){
if(response){
alert("We have successfully created a Facebook event with ID: "+response);
}
})
}
[/sourcecode]
And here is the source code of our events.php
[sourcecode language=”php”]
<?php
include_once("facebook-php-sdk/src/facebook.php");
define("FACEBOOOK_API_KEY","224818724242243");
define("FACEBOOK_SECRET_KEY","d57acde94xxxxc3d50a2x2yya9004476");
$name = $_POST[‘name’];
$token = $_POST[‘access_token’];
$startTime = $_POST[‘start_time’];
$endTime = $_POST[‘end_time’];
$location = $_POST[‘location’];
$description = $_POST[‘description’];
$fileName = "./me2.jpg"; //profile picture of the event
$fb = new Facebook(array(
‘appId’ => FACEBOOOK_API_KEY,
‘secret’ => FACEBOOK_SECRET_KEY,
‘cookie’ => false,
‘fileUpload’ => true // this is important !
));
$fb->setAccessToken($token);
$data = array("name"=>$name,
"access_token"=>$token,
"start_time"=>$startTime,
"end_time"=>$endTime,
"location"=>$location,
"description"=>$description,
basename($fileName) => ‘@’.$fileName
);
try{
$result = $fb->api("/me/events","post",$data);
$facebookEventId = $result[‘id’];
echo $facebookEventId;
}catch( Exception $e){
echo "0";
}
?>
[/sourcecode]
When we will invoke createEventWithPHP() javascript function, it will post all the event data to our PHP script. Then PHP script will create that event with Facebook PHP-SDK and print the newly created event id. Say for example, this newly created event id is 129312137169841, so we can go to http://facebook.com/events/129312137169841 and see how it looks with the profile picture
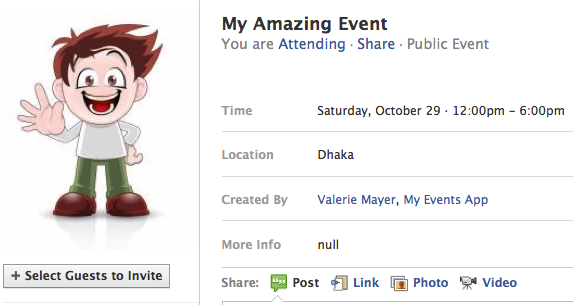
So thats for today, folks. I will be coming back with more of Facebook Events and Graph API in my next part. Stay Tuned.
Shameless Promotion 🙂 : By the way, if you are looking for a beautiful Admin Panel Theme for your PHP based projects/web-applications, you may want to check out Chameleon Circuit, which is developed by our Themio Team 🙂